Let’s start with explaining what go/golang is and why you should look at it if you are programming in any other language.
The most important thing: it is still a young tool. It’s only eight years old (since 2009). But it is used by the biggest brands like Uber, Netflix, Google. Therefore, we can assume that this language won’t be abandoned and learning it has a little more sense if you require something more than being hipster developer.
[toc]
Prerequisite
brew
is a small ruby script which allows you to install libraries with one command directly from command line. I use brew to install everything. You can read my previously note how to install brew before you start.- Gogland – IDE created by JetBrains. Somewhere, we have to write our code and run tests. In my opinion, you shouldn’t waste time trying using “just” code editors like Sublime or Atom.
Installation
The process of installation is very easy. First, what you have to do is to run that command:
brew install go
What’s next? Nothing, you can run Gogland (IDE) and start writing code.
Hello World
In my last notes, I’ve often talked about Test Driven Development. In this post, we use TDD, as well. This approach has more pros than cons.
Create file hello_world_test.go
. In go/golang each file with test scenarios must have suffix _test
.
package main
import (
"testing"
)
func TestHelloWorld(t *testing.T) {
if "Hello World" != HelloWorld() {
t.Fail()
}
}
To run tests, you can use command line by typing go test
in path with your project or using IDE by choosing option Run All
. In both cases, you should see something similar:
./hello_world_test.go:8: undefined: HelloWorld
FAIL _/Users/marcin/GoglandProjects/hello_world [build failed]
It’s red flag in TDD. Now, you have to create implementation.
Implementation
Create file hello_world.go
which contains:
package main
func main() {
}
func HelloWorld() string {
return string("Hello World")
}
Run tests again (go test
) and you should see this:
ok _/Users/marcin/GoglandProjects/hello_world 0.006s
Summary
Game Over. You are expert in go/golang. Now, you have your first TDD project, push it to GitHub.
Wait!
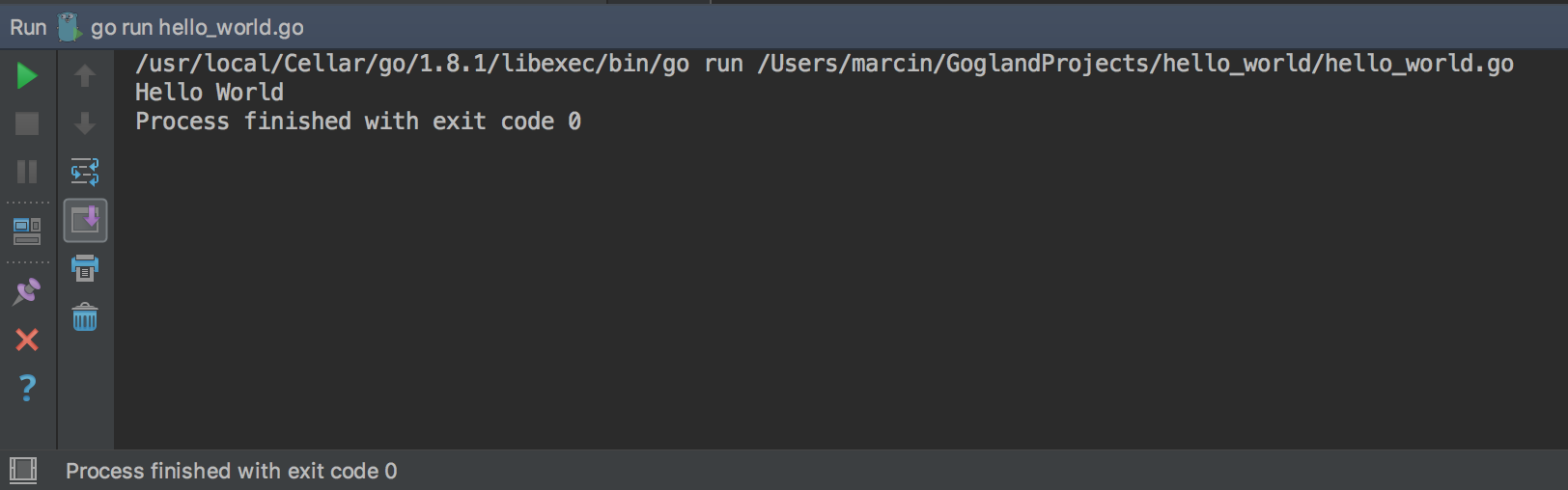
Someone could ask, did the author forget something? How to run the go/golang program? Ok, if you need it. Open your hello_word
again. Find there func main() {
and inside this function put this code:
fmt.Print(HelloWorld())
And before the function main
, paste that code:
import "fmt"
The whole file should look like bellow:
package main
import "fmt"
func main() {
fmt.Print(HelloWorld())
}
func HelloWorld() string {
return string("Hello World")
}
Now you can run your application by using run
in IDE or use command line with that command:
go run hello_world.go
As you assuming, the output will be:
Hello World